Alright, let’s talk about this “rob ruck” thing. It’s a fun little project I messed around with, and I figured I’d share how I got it done. It’s not rocket science, but hopefully, it’ll give you some ideas.
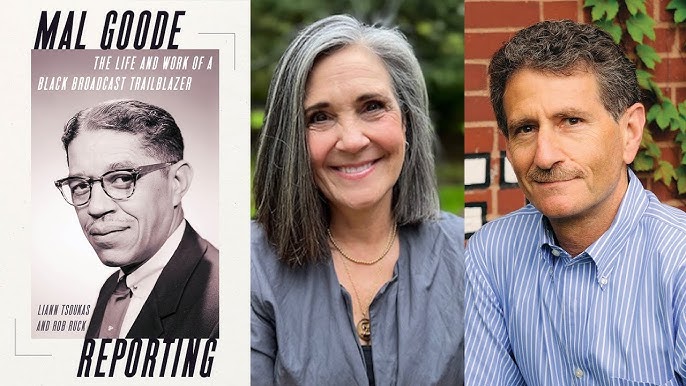
First Steps: Idea & Goal
So, what’s “rob ruck?” Basically, I wanted to automate grabbing specific data from a website – think product prices, sports scores, whatever. My goal was to set up a script that would run regularly, scrape the data, and then… well, I hadn’t decided exactly what to do with the data yet, but the scraping was the first hurdle. I thought i will start with a simple static web page.
Picking My Tools
I went with Python for this, ’cause it’s my go-to for quick scripting. Plus, it has some great libraries for web scraping. I settled on these:
- requests: To grab the HTML content from the website. Super easy to use.
- Beautiful Soup 4 (bs4): To parse the HTML and make it searchable. This is where the magic happens.
Installing them is a breeze with pip: pip install requests beautifulsoup4
. Done and dusted.
The Scraping Code
Here’s the basic flow of my script. I started pretty simple:
- Import the libraries:
import requests
andfrom bs4 import BeautifulSoup
. Obvious, but gotta say it. - Get the webpage: I used
*('the_url_i_needed')
to fetch the HTML. Big tip: always check the response status code! If it’s not 200, something went wrong. - Parse the HTML:
soup = BeautifulSoup(*, '*')
. This turns the messy HTML into a nice, searchable object. - Find the data: This is the tricky part. You gotta inspect the website’s HTML (right-click, “Inspect” in your browser) to figure out where the data you want is located. Is it in a
<div>
with a specific class? A<span>
with an ID? You gotta find the right CSS selectors.
For example, let’s say the price was in a <span>
tag with the class “price”. I’d use *('span', class_='price').text
to grab the text content of that tag. That class_='price'
thing? That’s just how Beautiful Soup handles classes (because class
is a reserved word in Python). I spent like half an hour figuring that out the first time. facepalm.
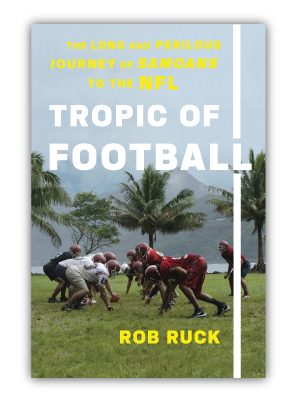
I used findall to find all elements then use a for loop to print the value. I used try and except for error handling.
Dealing with Dynamic Content (JavaScript)
Now, sometimes websites use JavaScript to load content dynamically after the page loads. If that’s the case, requests
and Beautiful Soup won’t cut it, because they only see the initial HTML. Then I found it needed a headless browser. That means something like:
- Selenium: This is a browser automation tool. It lets you control a real browser (like Chrome or Firefox) programmatically. You can tell it to wait for JavaScript to execute and then grab the rendered HTML.
Selenium is more complex than requests
and Beautiful Soup. You gotta install a WebDriver (like ChromeDriver) and configure it. But once you get it working, it’s powerful! But, thankfully i didn’t encounter it.
Putting it Together and Running
I wrapped all of this in a Python script. You can use a while loop to repeatedly load the page.
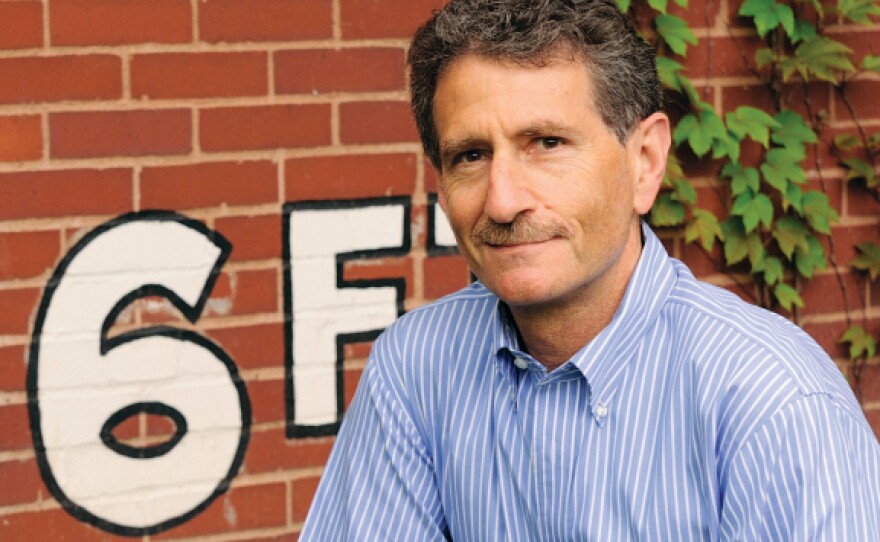
Next Steps: What to Do with the Data
Right now, my script just prints the scraped data to the console. Next steps include:
- Saving to a database: So I can track the data over time.
- Sending email alerts: If the price of something drops below a certain threshold.
- Visualizing the data: With charts and graphs.
But that’s for another day. For now, I’ve got a basic web scraper that works. And that’s the gist of my rob ruck project! Hope it was useful.