Okay, so let me tell you about this whole “encounter power fighting” thing I’ve been messing around with. It’s a bit rough around the edges, but I think it’s got potential.
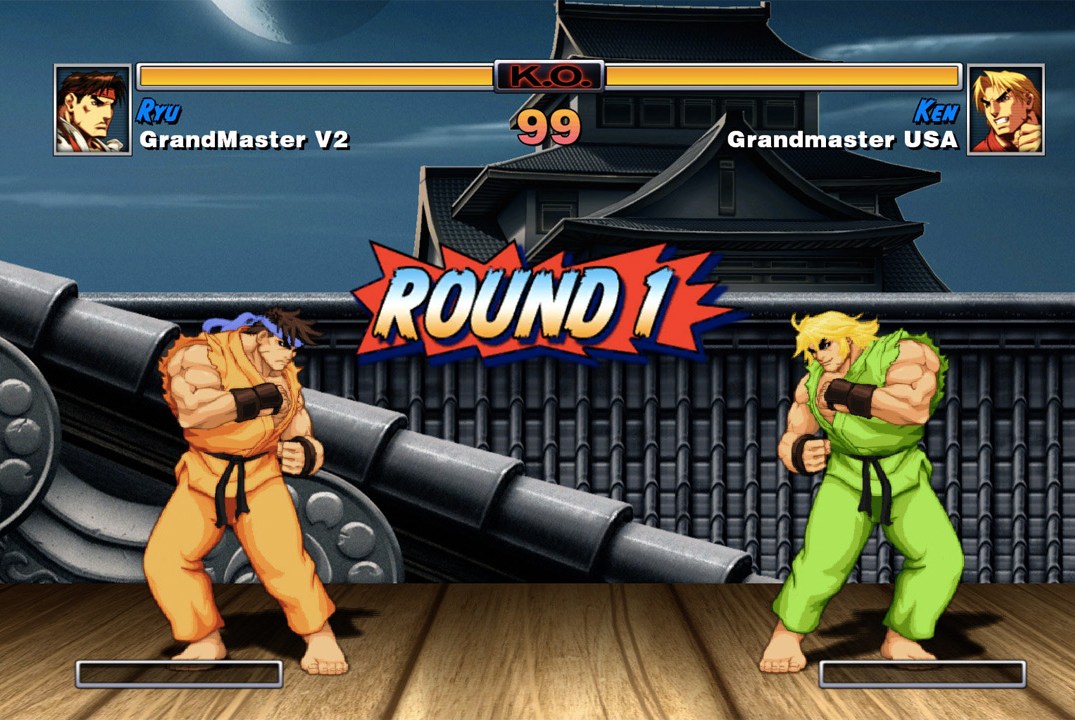
It all started when I was trying to figure out a way to, like, make a game mechanic where the strength of an attack depended on how the player encountered the enemy. Sounds kinda weird, right? But stick with me.
First, I just scribbled down a few ideas on a notepad. I’m talking like, really basic stuff. “Head-on = more power,” “From behind = critical hit,” that sort of thing. It was super disorganized, just a brain dump really.
Then, I decided to try prototyping it in Unity. I set up a super simple scene with a player character and a dummy enemy. Just cubes, nothing fancy. My initial thought was to use Unity’s collision detection. I added a `OnCollisionEnter` script to the player, trying to figure out the angle of impact.
csharp
void OnCollisionEnter(Collision collision) {
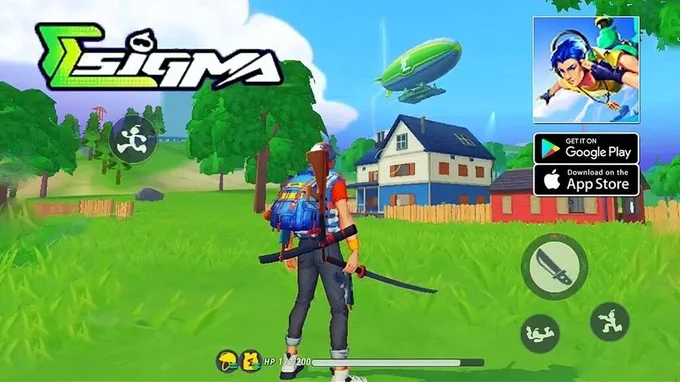
Vector3 direction = *[0].point – *;
float angle = *(*, direction);
*(“Angle: ” + angle);
This got me the angle, but it was kinda messy because the `*` can have multiple points and it felt kinda hacky to just use the `[0]` element.
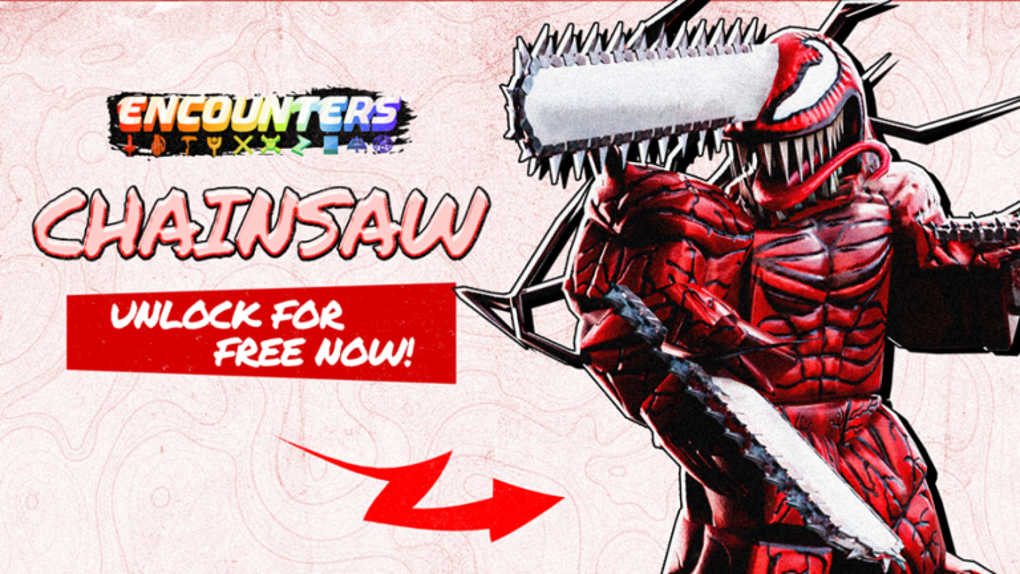
After that, I tried using raycasts. I attached multiple raycasts to my player character, each pointing in a different direction (forward, sides, back). When a raycast hit the enemy, I’d calculate a power modifier based on which raycast hit.
csharp
public class RaycastAttack : MonoBehaviour {
public float forwardPower = 1.0f;
public float sidePower = 0.5f;
public float backPower = 2.0f;
void Update() {
RaycastHit hit;
// Forward Raycast
if (*(*, *, out hit, 1.0f) && *(“Enemy”)) {
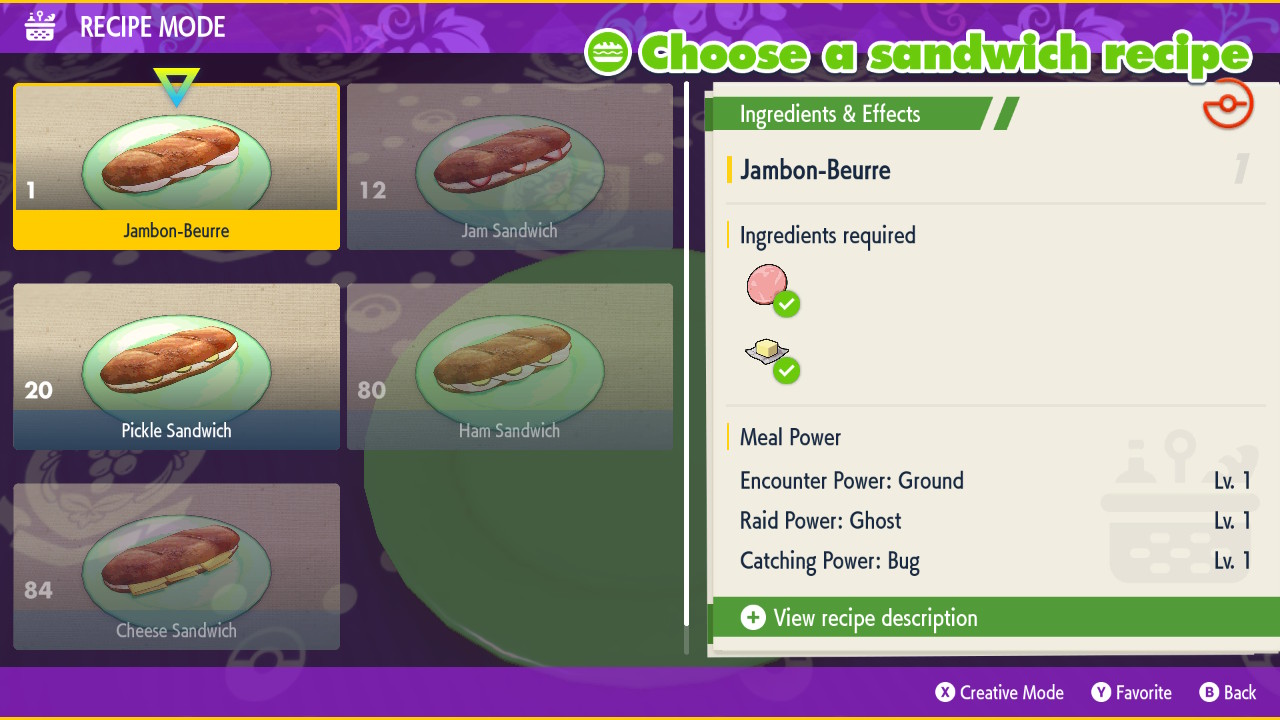
*(“Forward Attack! Power: ” + forwardPower);
// Side Raycasts (simplified)
// … similar code for left and right raycasts …
// Back Raycast
if (*(*, *, out hit, 1.0f) && *(“Enemy”)) {
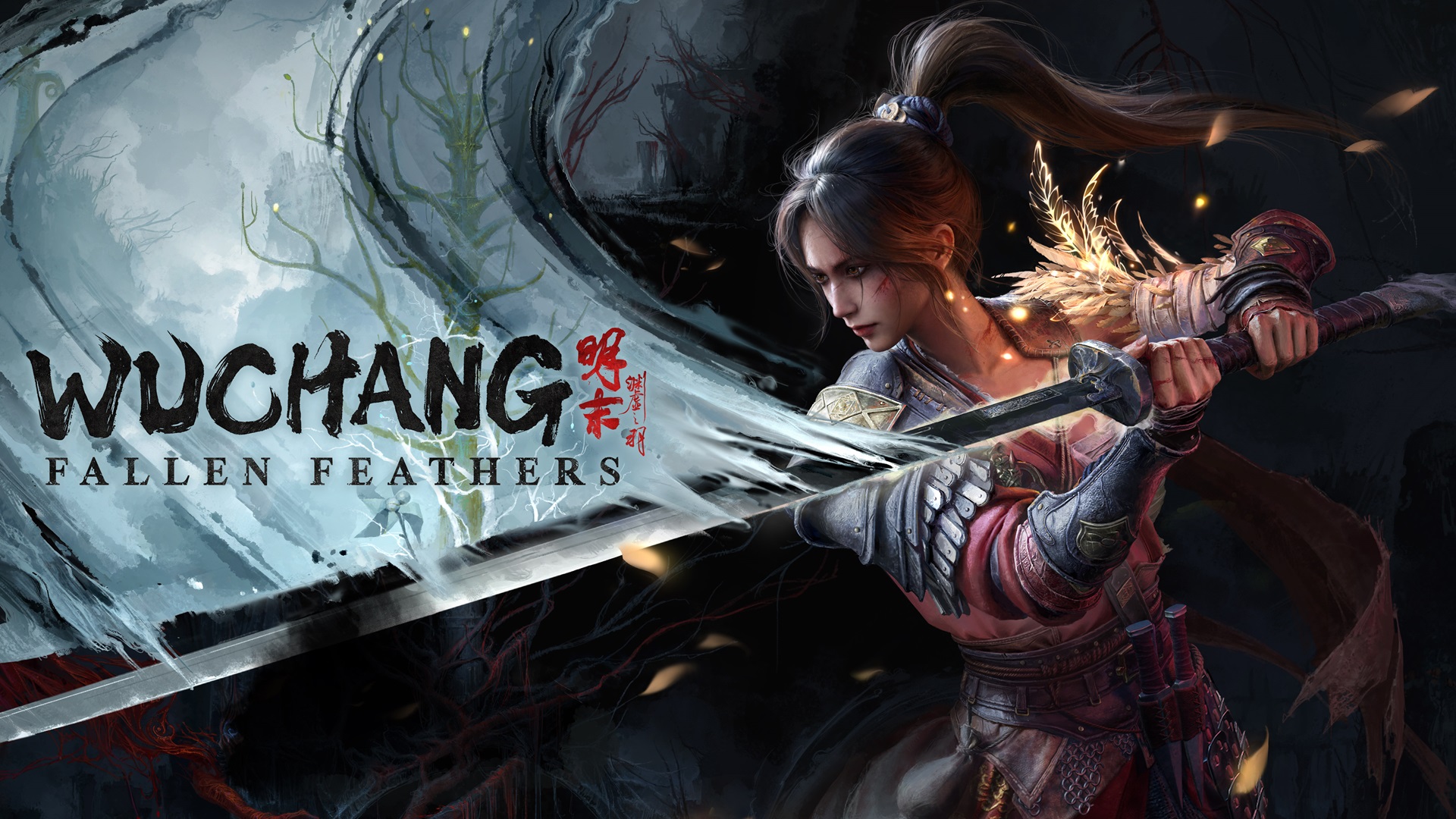
*(“Back Attack! Power: ” + backPower);
This felt better because I had more control, but it was still pretty clunky. I had to adjust the raycast lengths and positions manually, and it wasn’t very accurate. Plus, it looked kinda weird with all those raycasts constantly firing.
Finally, I landed on something that felt a bit more solid. I decided to define “attack zones” around the player character using empty GameObjects with `SphereCollider` components set to `isTrigger = true`. I positioned one in front, one on each side, and one in the back.
Then, in the player’s script, I checked which zones the enemy entered. Based on the zone, I applied a different power multiplier to the attack.
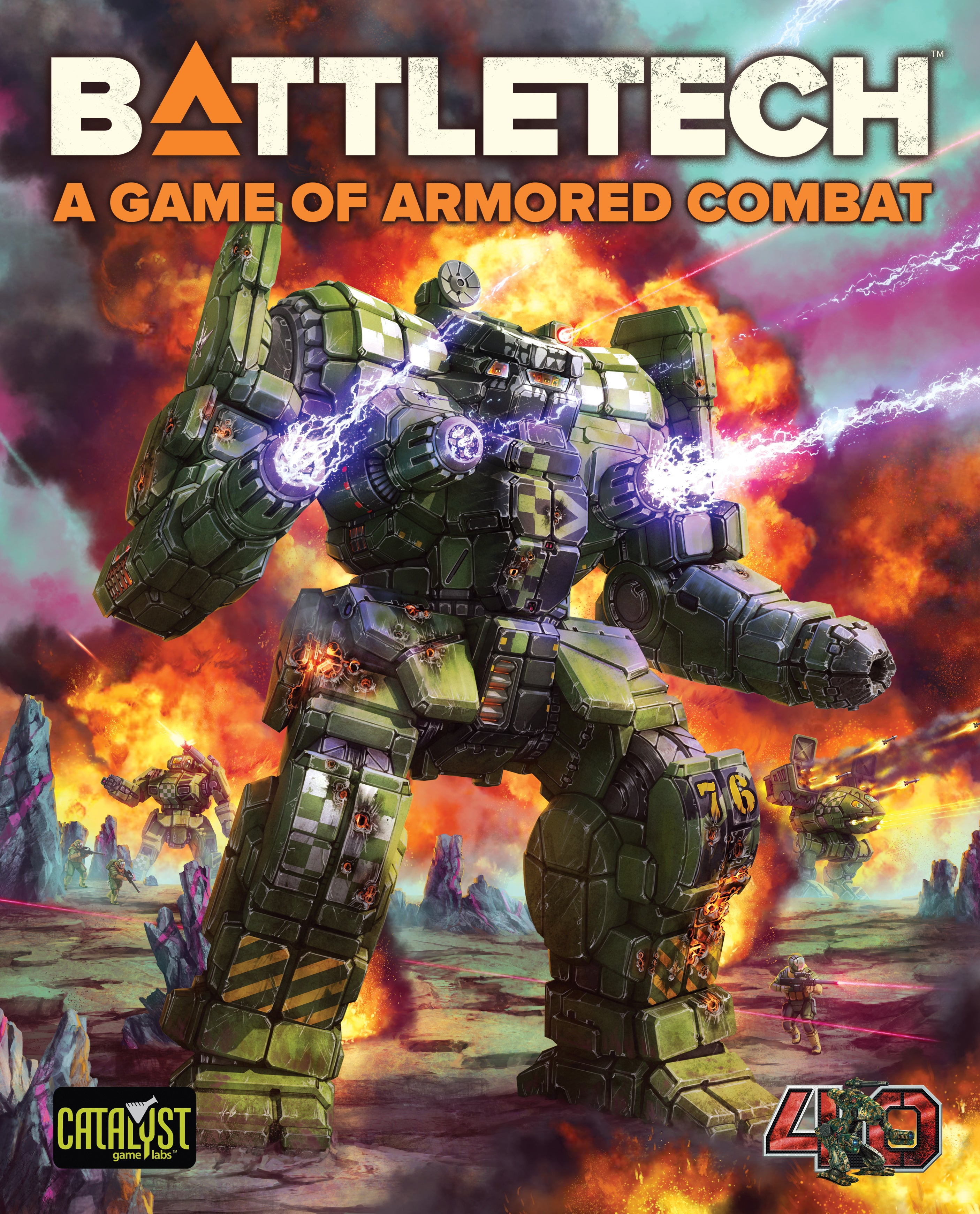
csharp
public class AttackZones : MonoBehaviour {
public float forwardPower = 1.0f;
public float sidePower = 0.75f;
public float backPower = 1.5f;
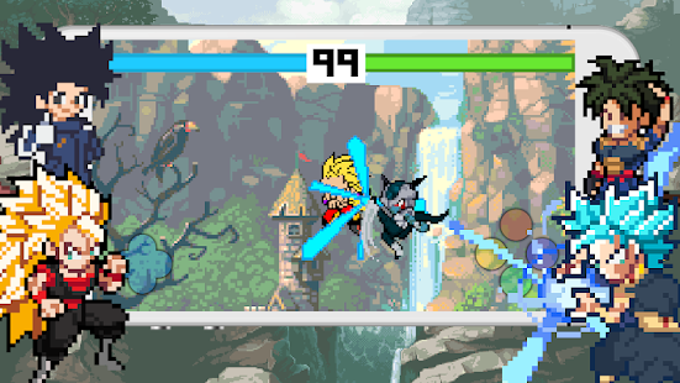
private string currentZone = “None”;
void OnTriggerEnter(Collider other) {
if (*(“Enemy”)) {
if (*(“Forward”)) {
currentZone = “Forward”;
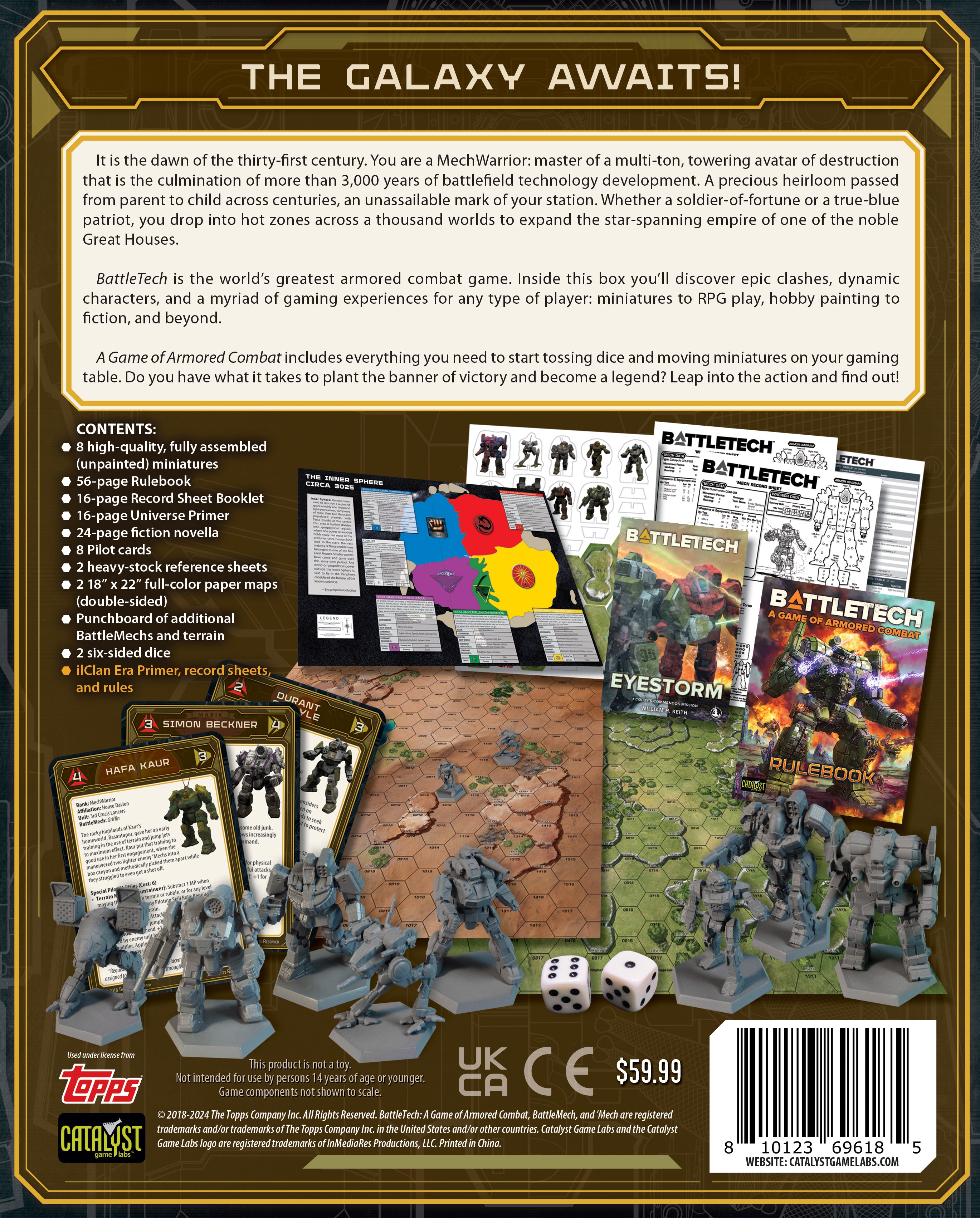
} else if (*(“Side”)) {
currentZone = “Side”;
} else if (*(“Back”)) {
currentZone = “Back”;
void OnTriggerExit(Collider other) {
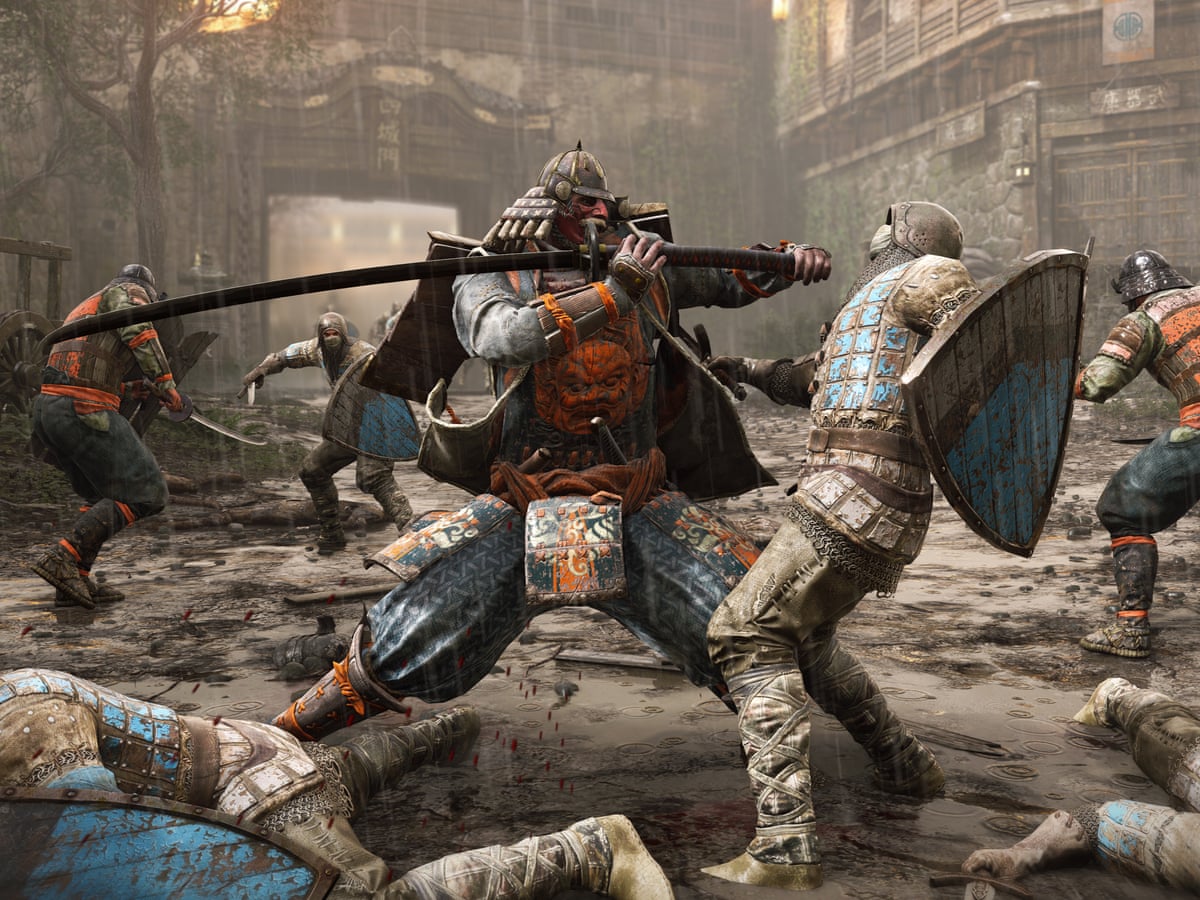
if (*(“Enemy”)) {
currentZone = “None”;
public float GetPowerModifier() {
switch (currentZone) {
case “Forward”: return forwardPower;
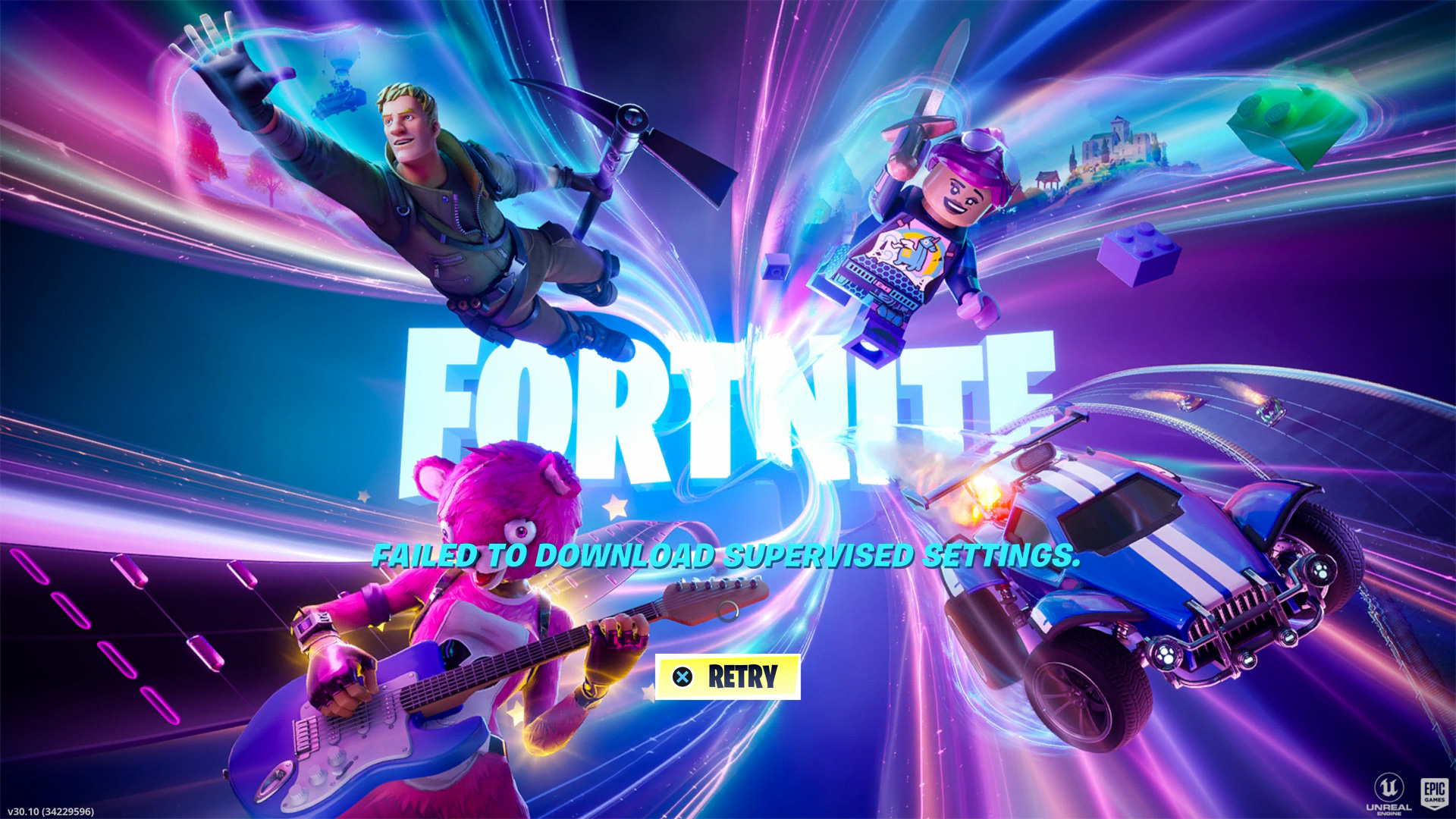
case “Side”: return sidePower;
case “Back”: return backPower;
default: return 1.0f;
This worked pretty well! It was relatively accurate, easy to adjust, and didn’t require any crazy raycast setups. The power modifier would then be passed to my attack function.
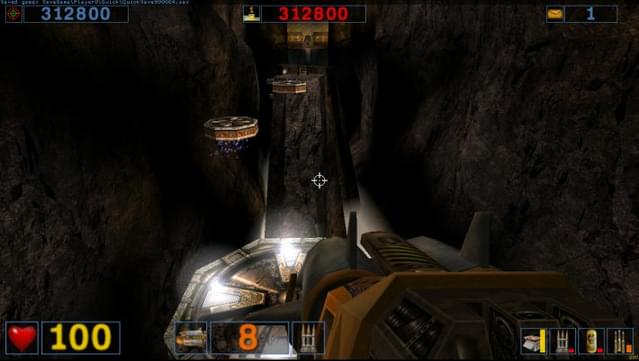
csharp
public void Attack() {
float powerModifier = *();
float finalDamage = baseDamage powerModifier;
*(“Damage Dealt: ” + finalDamage);
The biggest challenge was tweaking the sizes and positions of the attack zones to get the right feel. It took a lot of trial and error. I also had to add some extra checks to prevent the enemy from triggering multiple zones at once. And, of course, I needed to make sure the enemy had a proper `Tag` so my triggers would register properly.
But yeah, that’s basically how I tackled the whole “encounter power fighting” thing. It’s not perfect, and I’m sure there are better ways to do it, but it’s a start!